Benefits of using DAO design pattern.

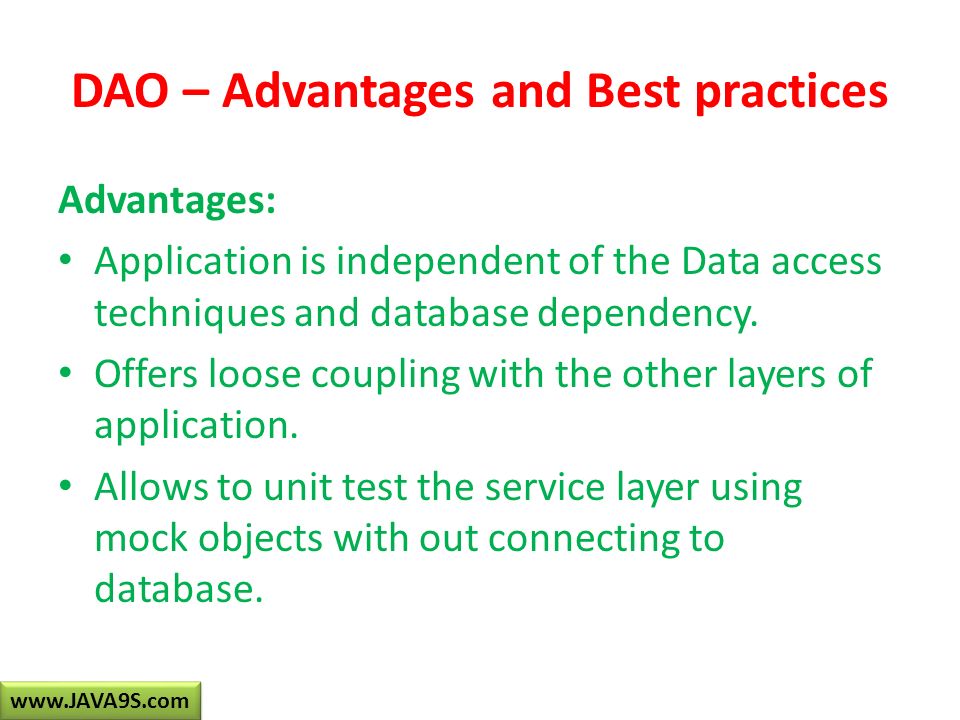

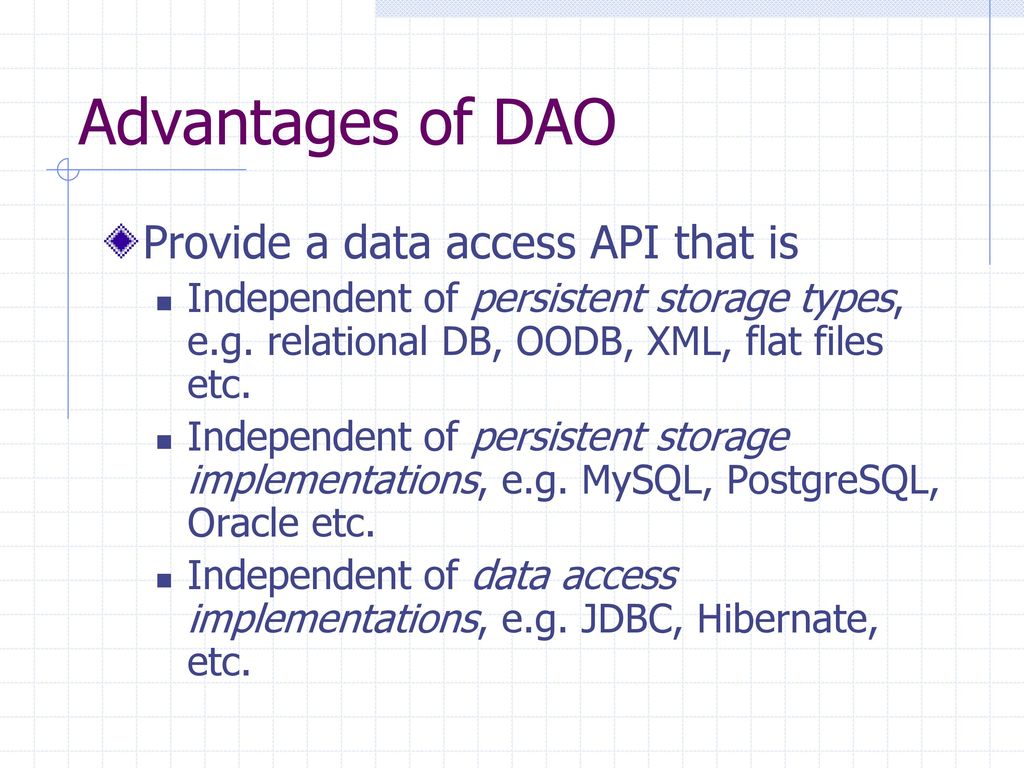
- DAO or Data Access Object design pattern is a good example of abstraction and encapsulation object oriented principles.
- It separates persistence logic is a separate layer called Data access layer which enables application to react safely to change in Persistence mechanism
- Servlet which is a controller may create a POJO, store data sent by the user in it and passes it on to the DAO. DAO queries the database and sends it back to theservlet and the servlet passes the data back to jSP again
- DAO is a class that usually has the CRUD operations like save, update, delete. DTOis just an object that holds data. It is JavaBean with instance variables and setter and getters. The DTO is used to expose several values in a bean like fashion.
- DAO pattern offers a logic to structure your persistence mechanism (the glue between your database and the model of your MVC).
- POJO stands for Plain Old Java Object, and would be used to describe the same things as a "Normal Class" whereas a JavaBean follows a set of rules. Most commonly Beans use getters and setters to protect their member variables, which are typically set to private and have a no-argument public constructor.

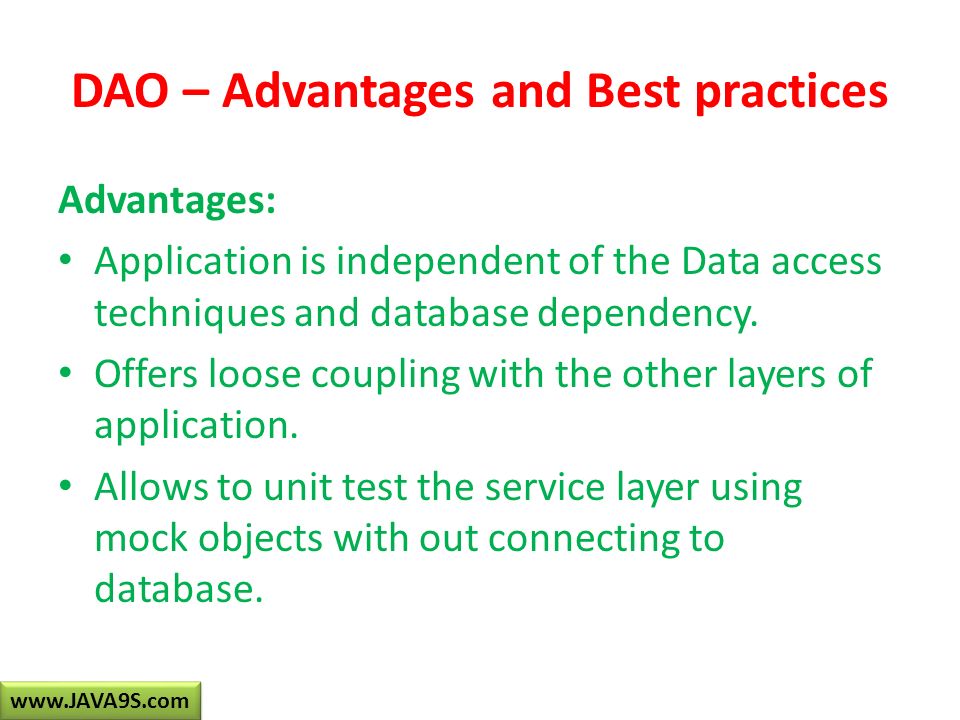

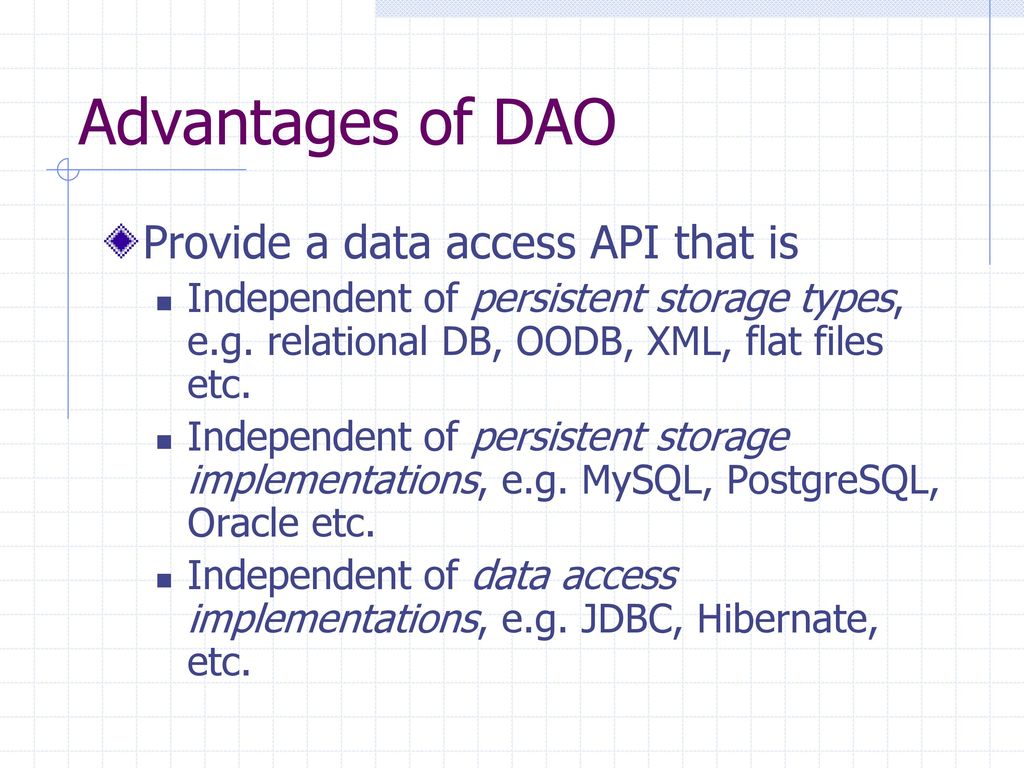